Introduction
In my previous article “Establishing a secure communication channel over HTTP” , I introduced a demo on how to exchange encrypted information over the HTTP protocol using RSA & AES algorithms.
This article provides an introduction to how to make encrypted communications between two endpoints over HTTP. The goal is to transmit data securely from one endpoint to another without the need for SSL/HTTPS connection.
For this purpose, we will take advantage of the Diffie–Hellman key exchange algorithm and the Advanced Encryption Standard (AES) algorithm.
The scenario in this article is that we have a desktop application that communicates with a web server on the internet. We want to ensure the information is securely exchanged, even if HTTPS is not present.
Before diving into the technical details, let’s have an overview on the Diffie-Hellman and AES algorithms.
The Diffie–Hellman Key Exchange Algorithm
Diffie Hellman (DH) key exchange algorithm is a method for securely exchanging cryptographic keys over a public communications channel. Keys are not actually exchanged – they are jointly derived. It is named after their inventors Whitfield Diffie and Martin Hellman.
The main purpose of the Diffie-Hellman key exchange is to securely develop shared secrets that can be used to derive keys. These keys can then be used with symmetric-key algorithms to transmit information in a protected manner. Symmetric algorithms tend to be used to encrypt the bulk of the data because they are more efficient than public key algorithms.
Does RSA Use Diffie-Hellman Algorithm?
Both RSA and Diffie Hellman (DH) are public-key encryption protocols used for secure key exchange. They are independent protocols that do not rely on one another. Unlike Diffie-Hellman, the RSA algorithm can be used for signing digital signatures as well as symmetric key exchange, but it does require the exchange of a public key beforehand.
What Is A Non-authenticated Key-agreement Protocol?
Authenticated Key Agreement protocols exchange a session key in a key exchange protocol which also authenticate the identities of parties involved in the key exchange. Anonymous (or non-authenticated) key exchange, like Diffie–Hellman, does not provide authentication of the parties, and is thus vulnerable to man-in-the-middle attacks.
Is Diffie-Hellman Algorithm Still Used Today?
DH is still in use today, but certain precautions have to be taken with respect to its building blocks. Authentication also needs to be implemented on top of DH to prevent man-in-the-middle threats.
Diffie–Hellman exchange by itself does not provide authentication of the communicating parties and is thus vulnerable to a man-in-the-middle attack. An active attacker executing the man-in-the-middle attack may establish two distinct key exchanges, one with Alice and the other with Bob, effectively masquerading as Alice to Bob, and vice versa, allowing her to decrypt, then re-encrypt, the messages passed between them.
How does the Diffie-Hellman key exchange work?
The following illustration highlights the process of performing DH key exchange:
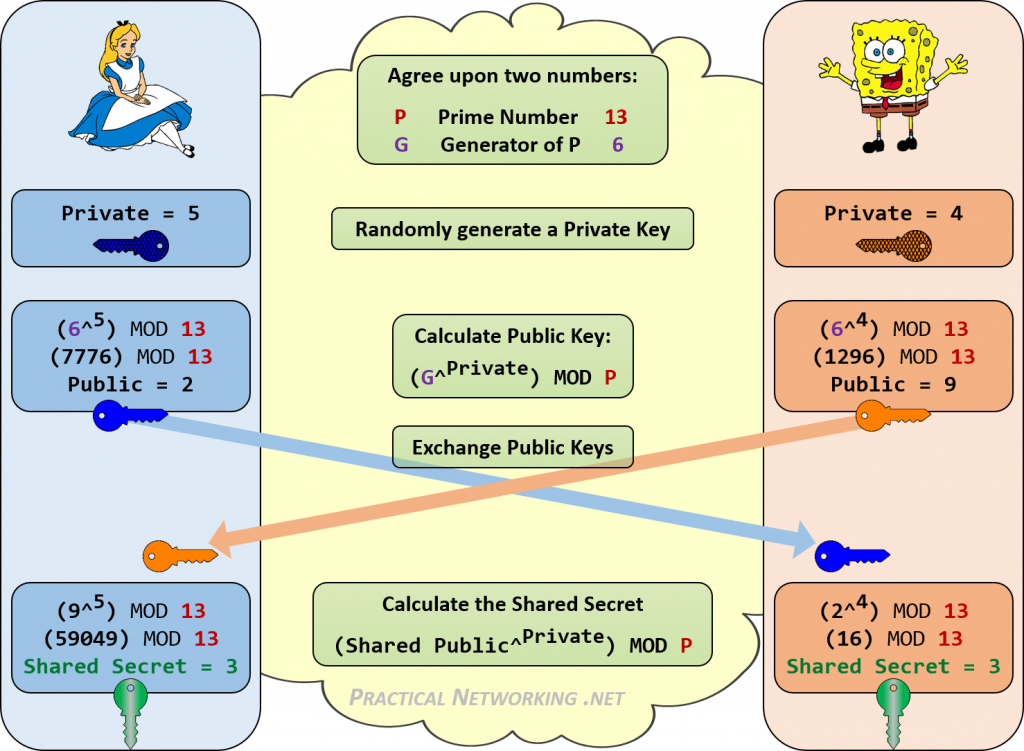
P: Large Prime
G: Non-zero Integer
Alice and Bob make the values of P and G public knowledge; for example, they might post the values on their web sites, so Eve knows them, too.
The Shared Secret can be used then to derive another key; for example, derive a symmetric key in a previously agreed algorithm.
Diffie-Hellman Algorithm Implementation
The scenario here is that we have a desktop application that communicates with a web server on the internet. We want to ensure the information is securely exchanged.
I made a very simple application using C# and PHP, which implement the session key generation and exchange. The idea is to provide you with insight to help you build a more complex version. The full source code of both client and server application are available the GitHub link:
https://github.com/iomoath/diffie_hellman_key_exchange
The client: Desktop Application (Written in .NET C# language)
The server: Web application (Written in PHP language)
The process:
- The client generates a unique session identifier to be used by the server identifying the client next time. (This part is better to be managed by the server).
- The client calculates: Large Prime number (P), Private Key and a Public Key.
- The client asks the server for it’s public key by providing the parameters: Prime Number, Client Public Key, Generator (G), and the Session Identifier generated in step-1
- The server uses the parameters received from step-3 to calculate: Server Public Key, Shared Secret and derive an AES key from the Shared Secret.
- The Server stores the AES key in it’s session management database and responds to the client with it’s Public Key.
- The client uses the server key to calculate the same Shared Key and derive the AES key
- At this point, the key exchange is complete. The client can now communicate with the server using the symmetric key (AES key) . The client must include its session identifier in any future communications so the server can identify the client AES keys from its session management database and be able to decrypt the data.
Server Application
The web application composed of two main files:
exchange.php: Responsible for handling DH key exchange requests.
functions.php: Contain core functions for DH algorithm implementation.
api.php: This is where the client will exchange information with the server after exchanging the session key through exchange.php
Web Application files:
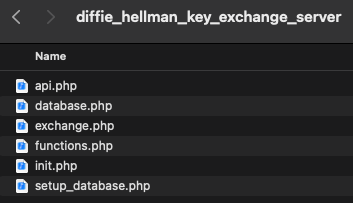
functions.php -> keycode() – Calculates the shared key and derive the AES key:
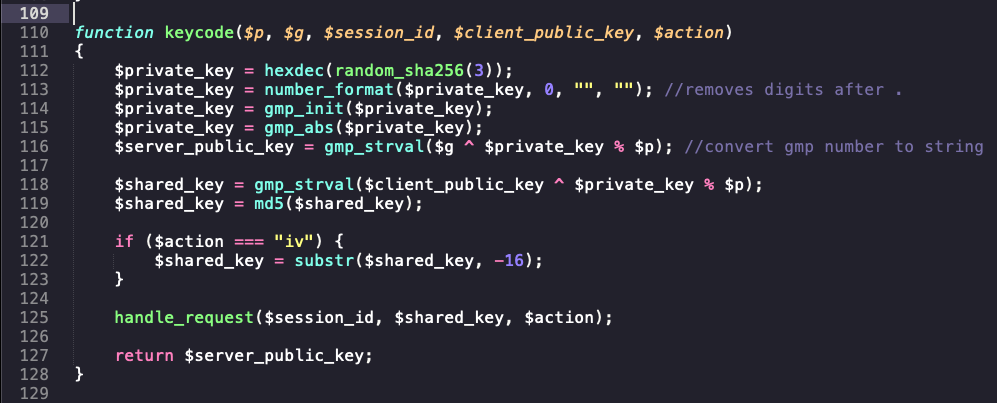
exchange.php:
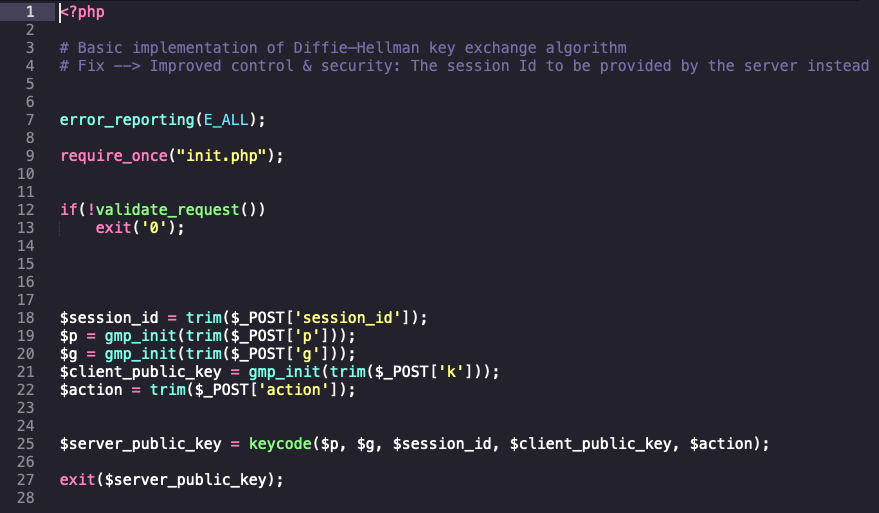
api.php
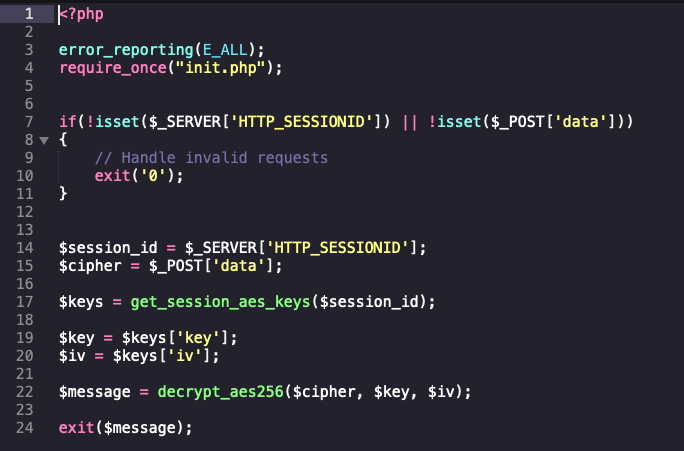
Client Application
The client application will attempt to establish an encrypted communication channel with the server and exchange some secret information.
The following code snippets are from the client application show key exchange function and information exchange:
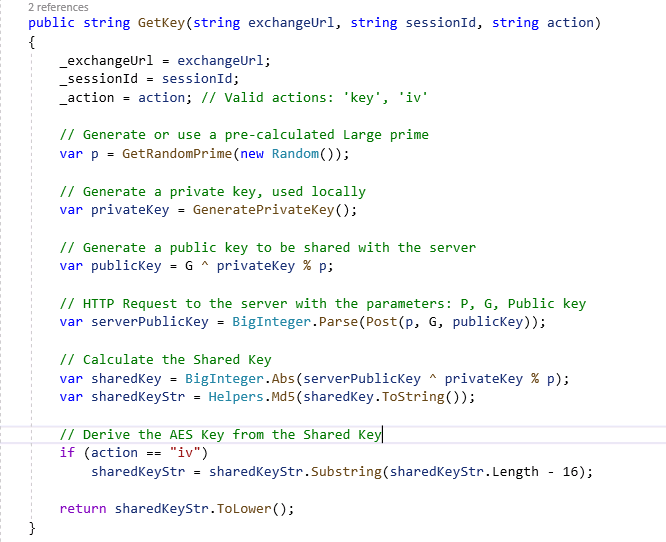
The following screenshot is the output of the client application. The application has performed two DH processes one for exchanging the AES Key and one for the AES (initialization vector) IV
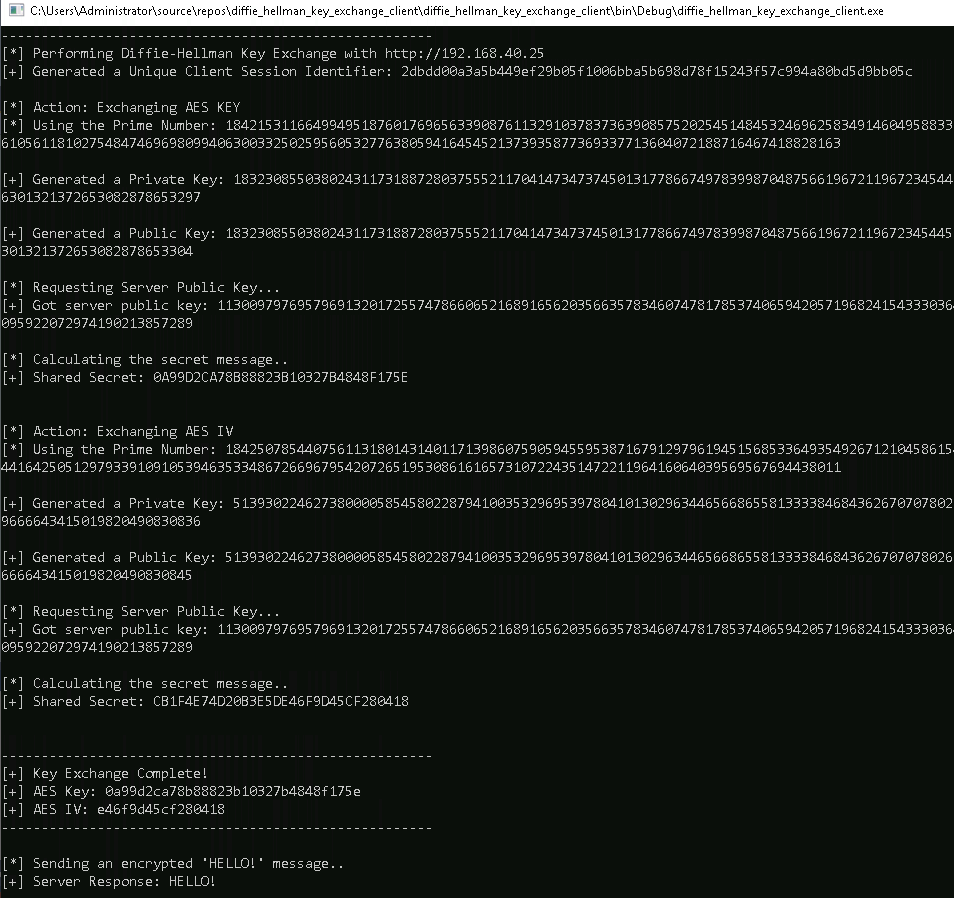
Inspecting HTTP Traffic
Let’s have a look on how the traffic generated by the client and server applications:

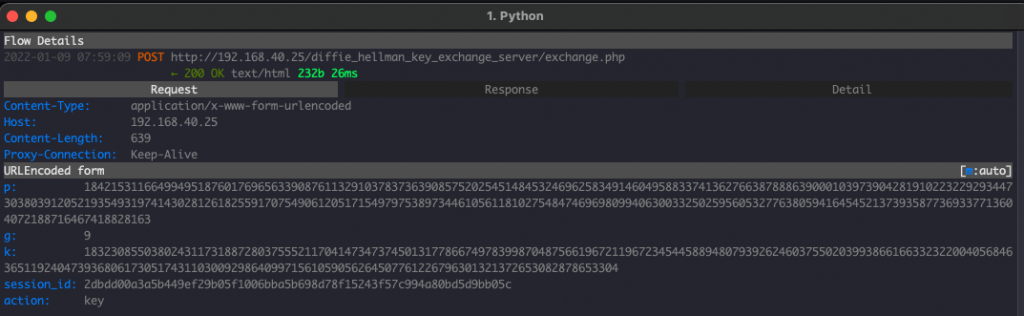
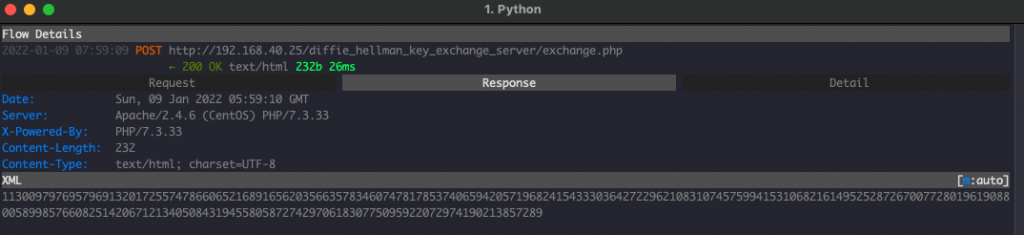
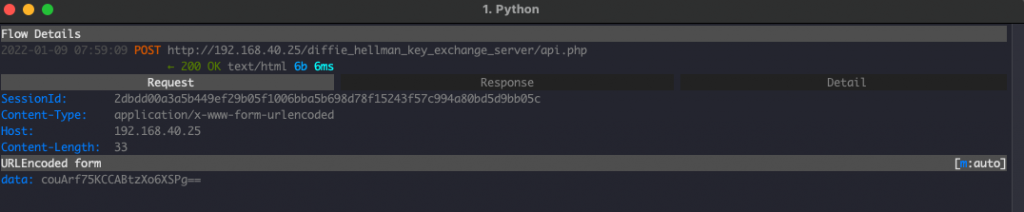
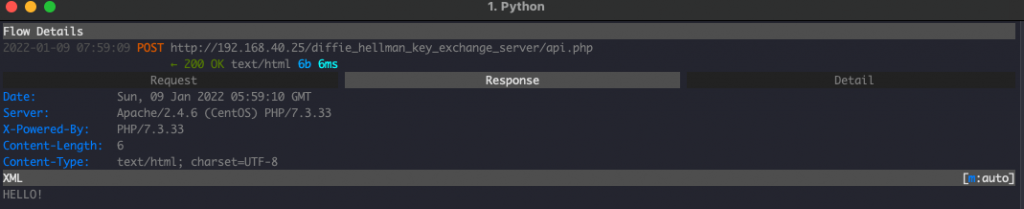
Considerations
The Diffie-Hellman key exchange algorithm does not provide authentication of the parties, and is thus vulnerable to man-in-the-middle attacks. Therefore Authentication needs to be implemented on top of DH.